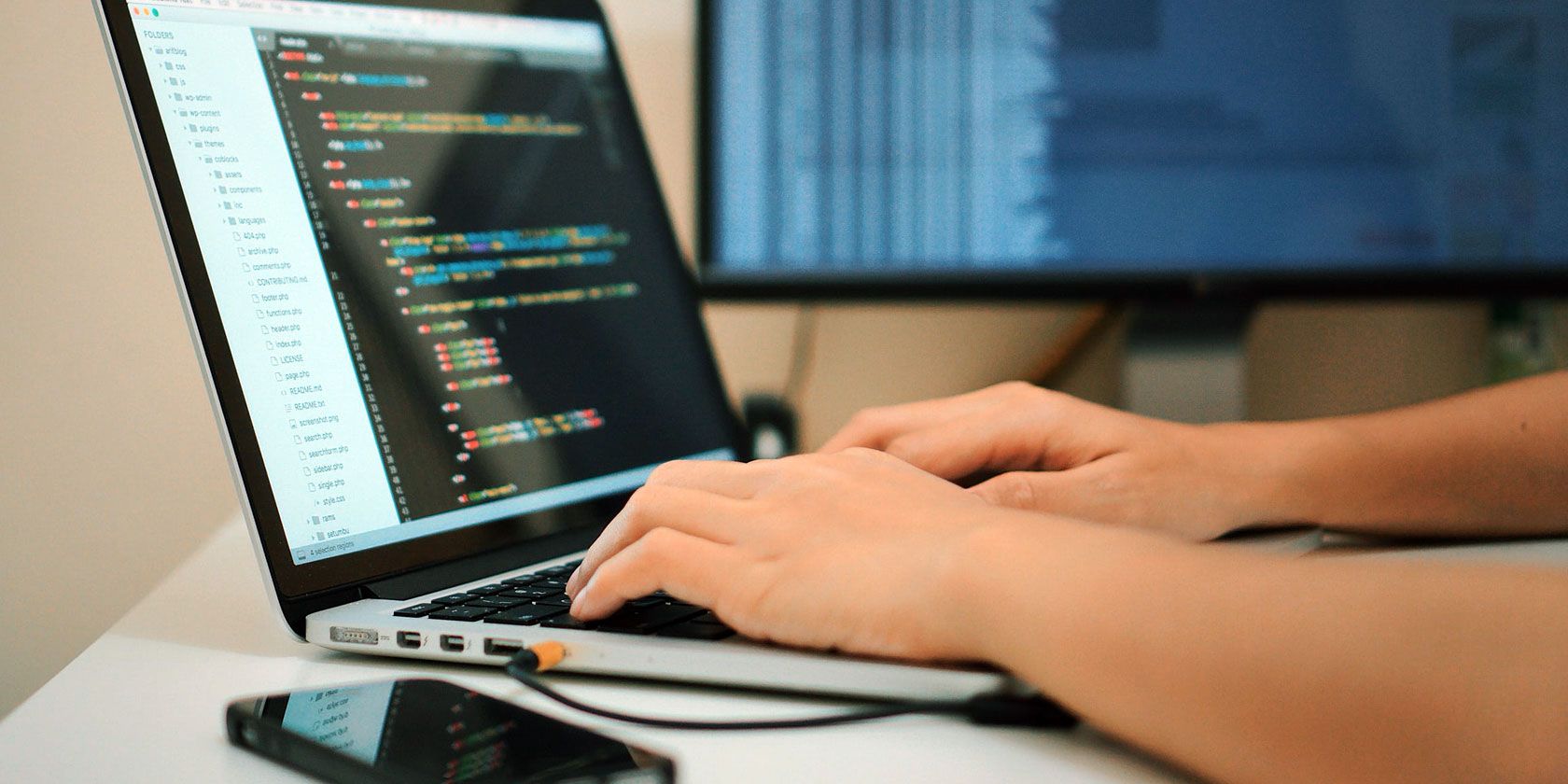
When you first begin to learn to program there are many things to study before you build your first app. Thinking like a programmer helps you break down problems into algorithms to solve them. Algorithms are the steps your code will take to solve a problem or answer a question.
It can be challenging if you’re a new coder to think like a programmer from the very start. Translating app ideas to actual code takes some practice.
To bridge the gap between what you want your app to do and the actual code you need to write, you can use pseudocode.
What Is Pseudocode?
Pseudocode is a plain-text description of a piece of code or an algorithm. It’s not actually coding; there is no script, no files, and no programming. As the name suggests, it’s “fake code”.
Pseudocode is not written in any particular programming language. It’s written in plain English that is clear and easy to understand.
While it’s not written in a programming language, there are still keywords used that refer to common coding concepts. These are written in uppercase letters to make it easier to read.
- START
- INPUT
- READ/GET
- PRINT/DISPLAY
- CALCULATE/DETERMINE
- SET
- INCREMENT/DECREMENT
- PROGRAM
- END
Here is a snippet of what pseudocode might look like for a program that asks you to input your favorite color and prints your choice.
START
PROGRAM getColor
Create variable Color
Ask the user for their favorite color
READ INPUT into Color
PRINT Color
END
This is a pretty simple algorithm written in pseudocode. Anyone can read and understand what this is trying to do. As the coder, all you have to do is bring this to life using whichever programming language you code in. Here’s the same program in JavaScript:
let color = window.prompt("What is your favorite color?");
console.log(color);
This program uses JavaScript syntax to write the algorithm. If you don’t know JavaScript it can be a little challenging to figure out what is happening.
Pseudocode writes the algorithm, programming languages write the syntax.
How Is Pseudocode Helpful?
Pseudocode helps you plan out your app before you write it. It helps you create algorithms in a format that is easier to read than code syntax. Once programming languages come into the picture it can be harder to understand what your code is doing.
The JavaScript example is easy to read if you know the language. But what if you’re just reading it and trying to determine the logic? Specific terms like window.prompt
or console.log
don’t reveal much about the algorithm.
Good software principles are important. If you interview to become a software engineer, they won’t expect you to memorize syntax. They will ask about your knowledge of algorithms and structure. You’ll write much better code if you construct your algorithms and structure before you start coding.
How to Write Pseudocode
Writing a full program in pseudocode requires a lot of different statements and keywords much like regular programming. In fact, once you get far enough along in your pseudocode it will start to look very close to a real program.
Let’s build on the keywords with pseudocode statements to build algorithms.
Conditionals
Conditional statements are critical to programming. These statements are IF statements or IF/ELSE statements which can add logic to your code. These statements are written in pseudocode using:
- IF
- ELSE
- ELSE IF
- THEN
Here’s a program that performs a simple IF/ELSE statement written in pseudocode. See if you can determine what this code is trying to do just by reading.
START
PROGRAM isOdd
Create variable Choice
Ask the user for a number
READ INPUT into Choice
IF Choice is even THEN
PRINT "No"
ELSE
PRINT "Yes"
ENDIF
END
It’s a pretty simple program. It asks the user for a number and does something depending on whether the number is odd or even.
Iteration
Another essential part of programming is iteration, also known as creating loops. Some common loops are for loops and while loops, both of which can be written in pseudocode.
START
PROGRAM forLoop
FOR 1 through 12
PRINT "Hello"
ENDFOR
END
This algorithm is for a program that will print “Hello” 12 times, which is a bit excessive but shows how simple it is to write a loop in pseudocode.
While loops are also written very easily
START
PROGRAM whileLoop
Create variable Counter
SET Counter equal to 1
WHILE Counter is less than 10
Print "Hello"
INCREMENT Counter
ENDWHILE
END
Another pretty simple algorithm using a while loop to print “Hello”. Both loop examples have a clear start and end to the iteration.
You also can write what is commonly known as Do-While loops. The keywords in pseudocode are different: REPEAT and UNTIL.
START
PROGRAM doWhileLoop
Create variable Counter
SET Counter equal to 1
REPEAT
Print "Hello"
INCREMENT Counter
UNTIL Counter is equal to 10
END
Just like a do-while loop, this will perform an action until certain criteria are met. Once it is met the loop will exit.
Functions
Functions are a programmer’s best friend. They contain code that can be called over and over again and are used in all high-level programming languages. Adding functions into your pseudocode is very easy.
START
PROGRAM sampleFunction
PRINT "This is a function"
END
You can call functions in pseudocode.
call sampleFunction
There is not much to functions; they’re very simple and you can add any logic you like.
Error Handling
Being able to write code that reacts to errors is very important when apps are developed. As such, you can include these catches into your pseudocode.
You can handle errors and exceptions using the keyword: EXCEPTION. Here’s a simple algorithm that catches an error
START
PROGRAM catchError
Create variable Number
Ask the user for a number
READ INPUT into Number
EXCEPTION
WHEN Number is not a number
PRINT "Error: Please pick a number"
END
The exception code will catch bad input from the user. Code testing is vital to writing good apps. Some of these exceptions will re-appear in your testing, so it’s good to be able to write them in your pseudocode when planning the app.
Software Development and More
Pseudocode is all about making you a better coder. Now that you know how to write it you can see just how useful it can be as part of your programming process. Programmers make some pretty good money, so if this is your career move you have a lot of opportunities if you learn a lot.
Knowing how to use pseudocode is recommended however you’re learning to code. Want to know more? Check out some basic principles that every programmer should follow.
Read the full article: What is Pseudocode and How Does it Make You a Better Developer?
Read Full Article
No comments:
Post a Comment